In this article, we will discuss how to create a Text-to-Speech tool using the Google Text-to-Speech API. We will run the Text-to-Speech API on Google AppScript, integrated with Google Sheets.
To start, head over to Google Cloud. If you haven’t activated your account yet, you can sign up for free. The Text-to-Speech API is also free to use within the usage limits, which are measured by the number of bytes of text converted.
Sign up Google Cloud
Make sure you’re logged in with your Google account, then click Start free.

When you sign up, you will receive $300 in free credits valid for the next 90 days.

For the payment part, you are required to enter your payment profile and credit card information. However, don’t worry, you won’t be charged until you activate your full account.
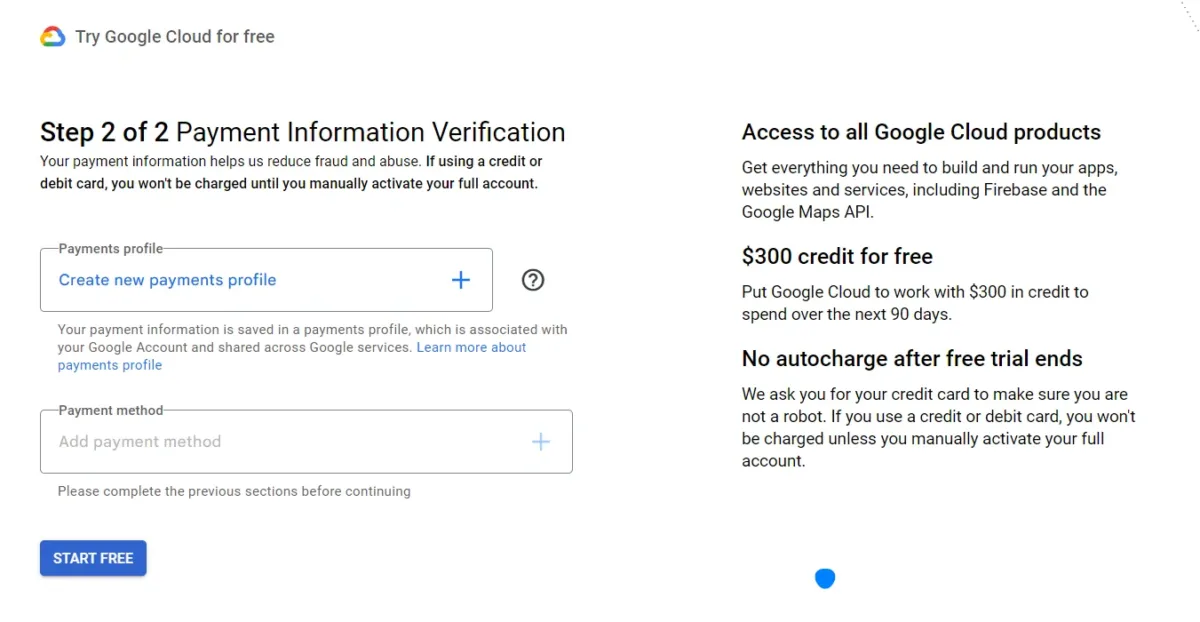
After completing the signup, you will be redirected to the Google Cloud home screen, where a default project named “My First Project” will be automatically created. Take note of the dropdown box on the top menu bar, right next to the Google Cloud title. This dropdown box allows you to select the active project. You can click on it to create a new project, but for this article, I will use the default project.
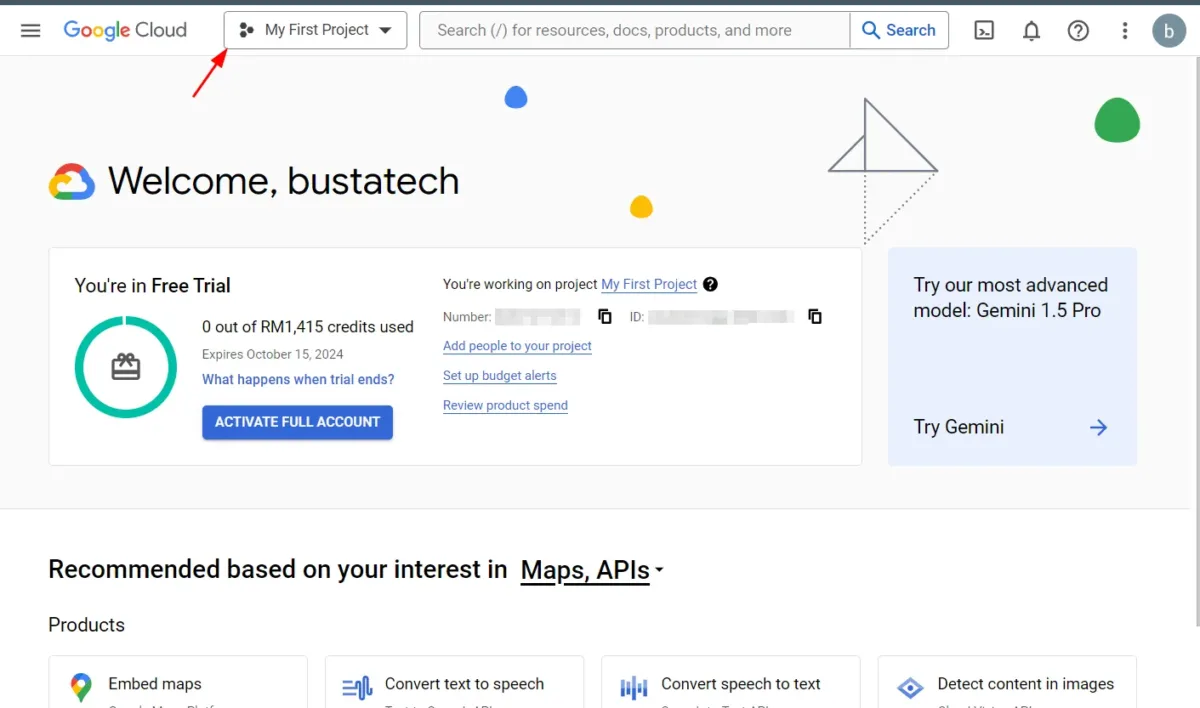
Google Cloud Project Configuration
The first step is to rename the project to something more descriptive. Click on the three-dot icon in the top right corner and navigate to “Project settings.”

Rename the project and click SAVE.

After that, navigate to the top left corner button, then go to “APIs & Services” > “Enabled APIs & services.”

Click the “ENABLE APIS AND SERVICES” button.

Search for ‘text to speech’ and click on the Cloud Text-to-Speech API.


On the Cloud Text-to-Speech API page, you can review the detailed pricing. As mentioned earlier, conversions are free as long as they do not exceed 1 million characters. Please note that the character limits vary for WaveNet voices, Standard voices, and Studio voices. Also, be aware that these policies may change periodically.

To proceed, click on the “ENABLE” button.

After enabling the API, the next step is to create credentials. This will generate an API key that allows your script to use your Google account to access the API. Head over to the top left button, then go to “APIs & Services” > “Credentials.”

On the Credentials page, click on “CREATE CREDENTIALS” > “API Key”:
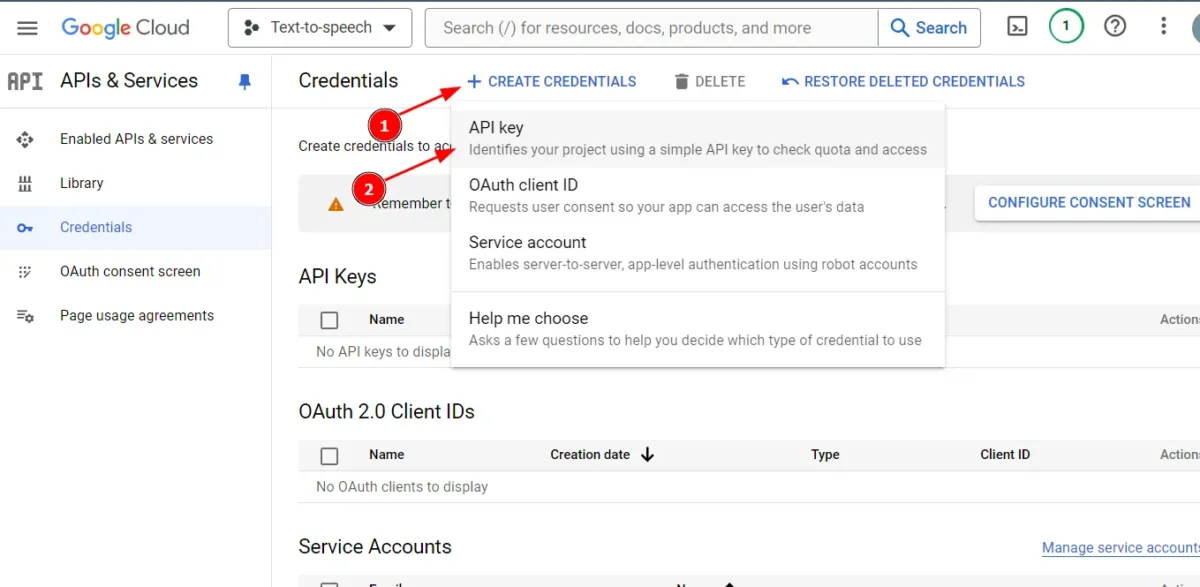
An API key will be generated. Remember not to share this key with anyone as it provides access to the API under your Google Account. Copy this API key and store it securely.

Google Apps Script on Google Sheet
Create a new Google Sheet file and place it in a folder where you intend to store generated audio files. In Google Sheets, navigate to “Extensions” > “Apps Script”:

This will open a new Apps Script window. This script is directly associated with the Google Sheet you created earlier. You can rename the Apps Script project for clarity.
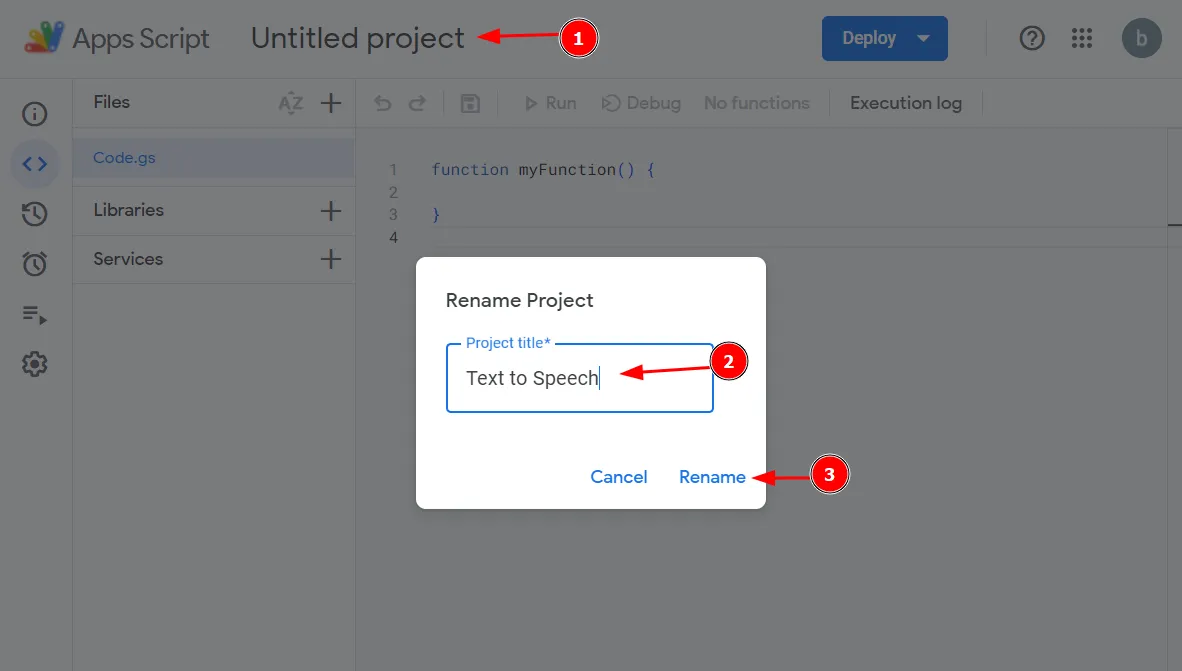
Now, let’s proceed with the script. Below is the complete code with explanations for each section:
function tts() {
// prepare the url for the API
var api_key = '<insert your API key here>';
var url = 'https://texttospeech.googleapis.com/v1/text:synthesize?key=' + api_key;
// prepare payload for the conversion
var payload = {
input: { text: 'hello, how are you?' },
voice: { languageCode: 'en-US', name: 'en-US-Journey-D' },
audioConfig: { audioEncoding: 'MP3', speakingRate: '1.00' }
};
// triggering the API for the conversion
var options = {
method: 'post',
contentType: 'application/json',
payload: JSON.stringify(payload)
};
var response = UrlFetchApp.fetch(url, options);
var responseJson = JSON.parse(response.getContentText());
var audioContent = responseJson.audioContent;
var blob = Utilities.newBlob(Utilities.base64Decode(audioContent), 'audio/mp3', 'output.mp3');
// open the folder to save the file
var folder = DriveApp.getFolderById('<insert your folder id here>');
// save the file to the folder
folder.createFile(blob);
}
Important note:
- Line 4: Insert your API key into this line.
- Line 26: Insert your Google Drive folder ID here. The script saves the generated audio file to this folder. To find the folder ID in Google Drive, open the folder and extract the ID from its URL (the part after the last “/”). Ensure you have write access to this folder to avoid errors when saving the output file.

Explanation:
- Lines 3-5: Prepare the API URL with placeholders for the API key.
- Lines 7-8: Set the text to be converted (“hello, how are you?”). You can also configure the voice and audio settings such as speaking rate here.
- Lines 14-23: Trigger the API for text conversion and save the resulting MP3 file.
- Lines 25-26: Save the MP3 file to the specified folder in Google Drive.
After replacing the API key and folder ID, your script should look like this:
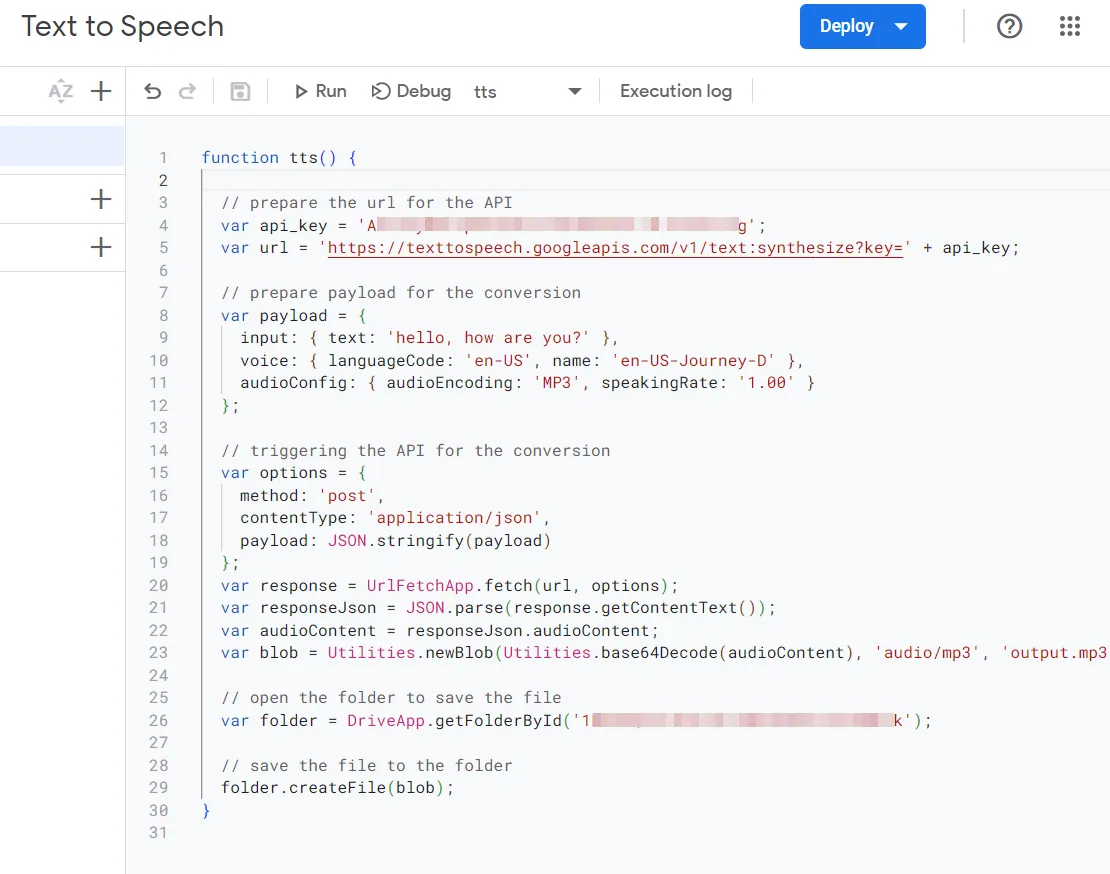
Once your function is ready, you can run it by clicking the “Run” button. If you have multiple functions, ensure that “tts” is selected.

The first time you run the function, it will request permission to access your data because it saves audio files to your Google Drive folder. Proceed by clicking “Advanced” and then “Go to Text to Speech (unsafe).”
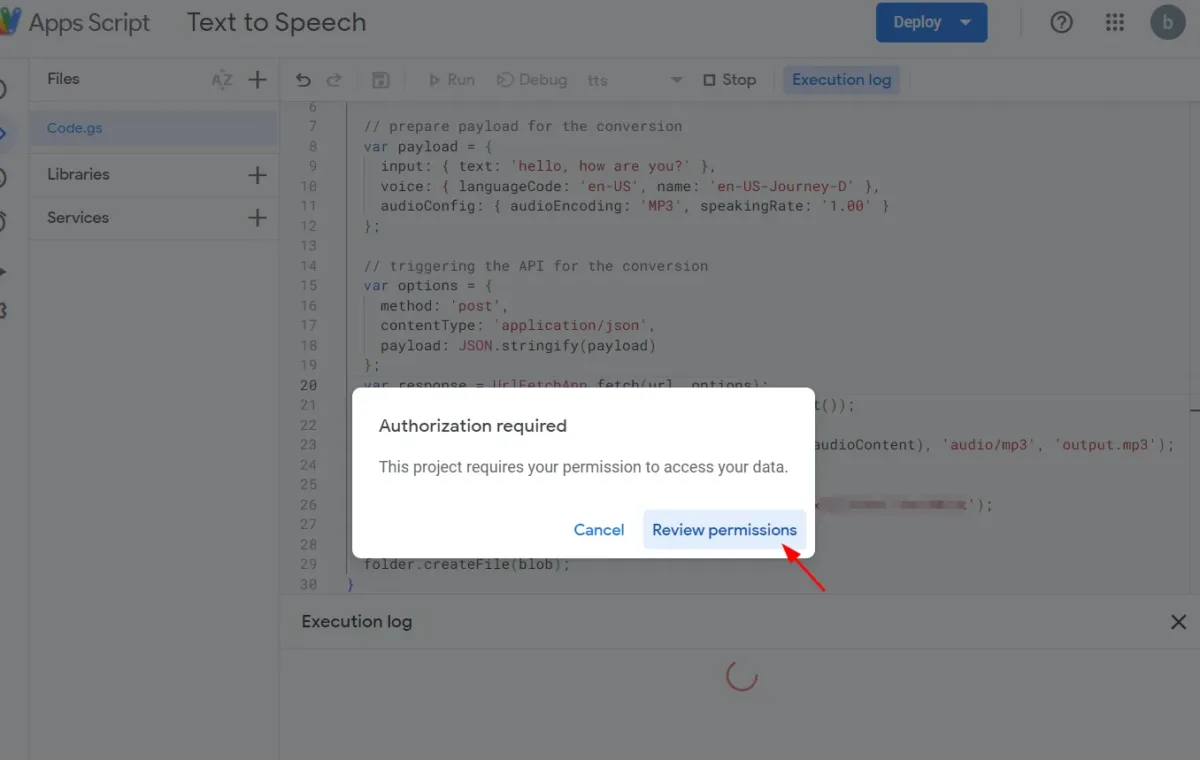

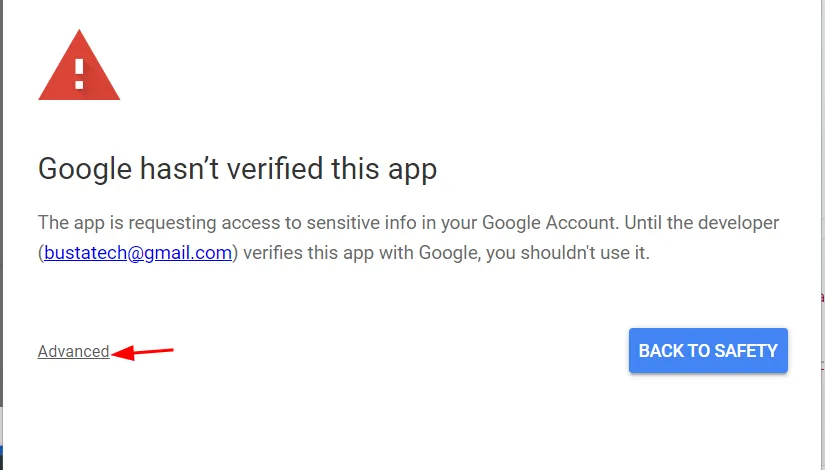


If configured correctly, the Execution Log should show “Execution started” and “Execution completed.” You should also find a new “output.mp3” file in your project folder on Google Drive.



Improvement to Make it More Useful
Now that the script is functional, we can enhance the utility of Google Sheets by making text conversion dynamic. We’ll begin by editing the Google Sheet to include text input and display the output URL. Additionally, we can integrate configuration options directly into the sheet.

I’ve designed a simple form where users can input text, select a voice for conversion, and adjust the speaking rate. For a comprehensive list of available voices, please refer to this link.

For voice selection, you can create a dropdown menu containing your preferred list of voices.

For the Generate button, create a Drawing and add a button.
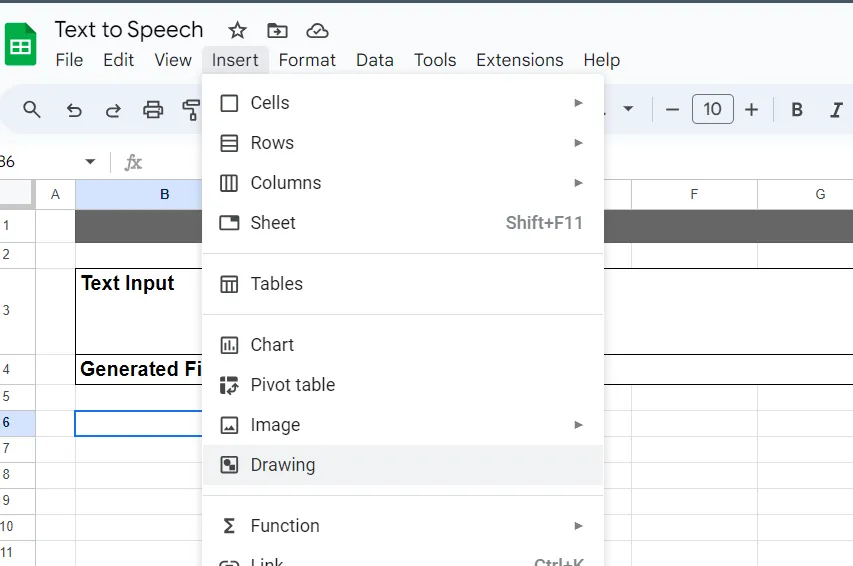

Assign the script function to this button by selecting it, clicking the three dots for more options, and choosing “Assign script.” Enter the function name (e.g., “tts”) and click “OK.”


Next we update the script to make use of all the information from the Google Sheets.
function tts() {
// read all information from the form
var sheet = SpreadsheetApp.getActive().getActiveSheet();
var text_input = sheet.getRange('C3').getValue();
var voice = sheet.getRange('C4').getValue();
var speaking_rate = sheet.getRange('G4').getValue();
// prepare the url for the API
var api_key = '<insert your API key here>';
var url = 'https://texttospeech.googleapis.com/v1/text:synthesize?key=' + api_key;
// prepare payload for the conversion
var payload = {
input: { text: text_input },
voice: { languageCode: 'en-US', name: voice },
audioConfig: { audioEncoding: 'MP3', speakingRate: speaking_rate }
};
// triggering the API for the conversion
var options = {
method: 'post',
contentType: 'application/json',
payload: JSON.stringify(payload)
};
var response = UrlFetchApp.fetch(url, options);
var responseJson = JSON.parse(response.getContentText());
var audioContent = responseJson.audioContent;
var blob = Utilities.newBlob(Utilities.base64Decode(audioContent), 'audio/mp3', 'output.mp3');
// open the folder to save the file
var folder = DriveApp.getFolderById('<insert your folder id here>');
// save the file to the folder
var file = folder.createFile(blob);
// update url to the form
sheet.getRange('C5').setValue(file.getUrl());
}
Explanations for the addon codes:
- Lines 3-7: Retrieve text input, voice selection, and speaking rate from Google Sheets and store them in variables.
- Lines 13-18: Use the dynamically fetched values for text, voice name, and speaking rate instead of static values.
- Lines 34-38: Save the URL of the generated file to cell C5 in Google Sheets.
When you use the Generate button, it may request permission again due to script changes and additional permissions needed. Follow the prompts to grant the required permissions.
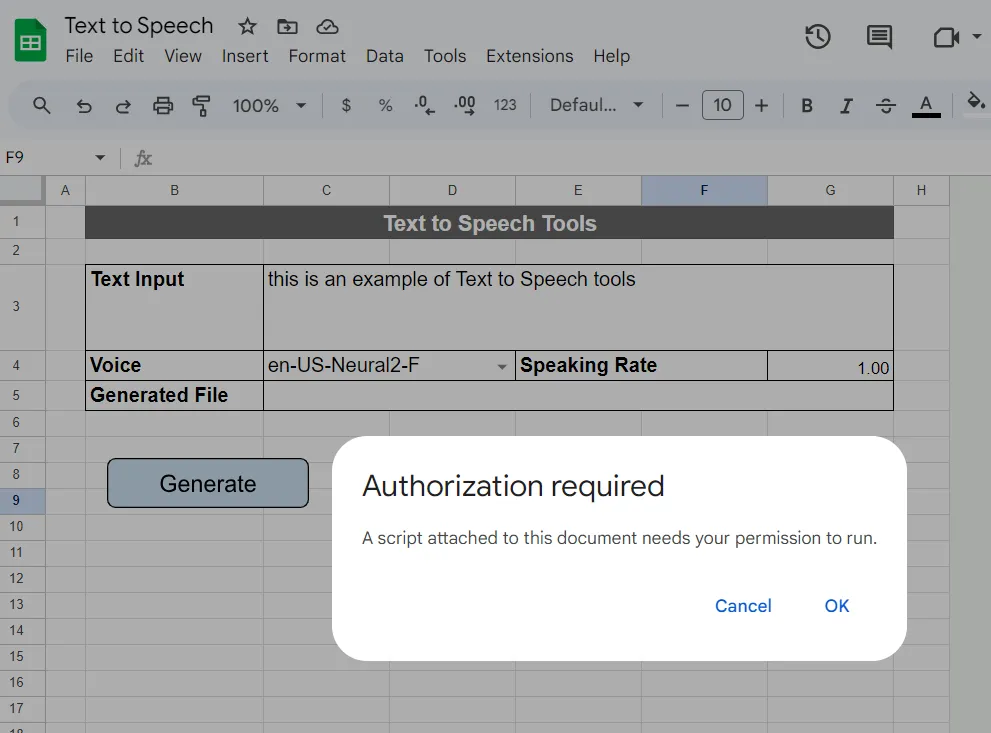
Once permissions are updated, rerun the script using the Generate button. This time, it should automatically convert the text to speech. The URL to access the generated file will update in the form, allowing you to click it and open the file directly.

I hope this article helps you understand and implement Google Sheets integration with the Text-to-Speech API. Note that charges may apply if you exceed the free tier usage. Thank you for reading!